Crowdbotics models, how to edit, and how to build with the model editor.
Getting Started with the Crowdbotics Model Builder
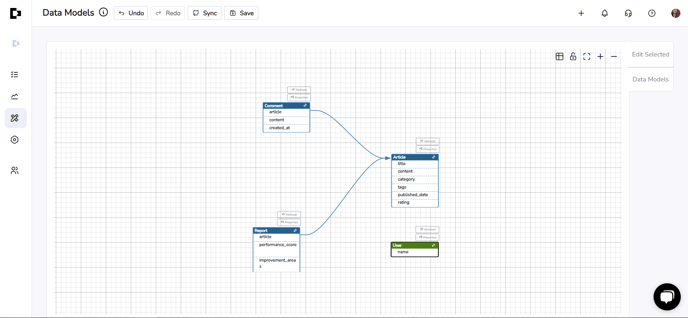
All apps have a frontend and a backend. The frontend is what the user sees, interacts with, and experiences (buttons, colors, fonts, stuff like that). The backend is everything going on behind the curtain. The Crowdbotics Model Builder is for building backends.
You might’ve heard of an API before, and it’s important for backends, so here’s what it is and how it works: API stands for Application Programming Interface, and it is a way for information from one program to be shared with other programs.
Imagine you’re at a fast food restaurant. You can’t get your food until you order, and you have to order through the cashier. Think of the cashier as an API. The API takes your order, reports it to the kitchen, and the kitchen makes your food.
When you have a program on your laptop, it can do a lot of digging around and talking to parts of your laptop. However, it cannot go over to LinkedIn and mess with data there, because just like the restaurant doesn’t want you in the kitchen, LinkedIn doesn’t want your programs in their data. So LinkedIn offers an API: a menu for ordering specific stuff. The program on your laptop makes an order through LinkedIn’s API and LinkedIn fulfills the order.
Now you’re getting the hang of APIs.
Crowdbotics’ new Model Builder makes creating these APIs very simple. You don’t need to learn Django and you don’t need to use Firebase. You can just use the CB Model Builder.
How to use the Crowdbotics Model Builder
Terminology
Before proceeding, let's define a few key terms.
Model:
A model refers to a grouping of data you want to keep track of, usually stored in a table.
For example, if you are building a marketplace app and you want to know and store information about each transaction that takes place, you could call your model “bill” or “transaction”.
Then, each field (defined below) within this transaction model would have pieces of information (data) that you want to know about each transaction in your marketplace. Generally, each model maps to one database table.
Field:
Fields are pieces of data that pertain to a model, or, more simply, characteristics of a model. Continuing the marketplace example, some fields you would want to keep track of might be a transaction’s total, transaction number, buyer, seller, and date.
Type:
Refers to what type of data this field is. For example, if the name of the field is “quantity,” you would want that piece of data to be an integer and not a character or URL.
Basic data types include integers, floating point numbers, characters, and booleans, which are respectively listed as IntegerField, FloatField, CharField, and BooleanField on the CB App Builder.
As shown above, there are a number of other options for field types. Most of them, like the DecimalField and DateTimeField are self-explanatory, but others like OneToOneField require explanation.
A OneToOneField is a field that links to another model. For example, you can create a User model that contains the fields name, email, phone number, and review (shown below). While the name, email, and phone number are simple field types like the ones mentioned above, the review field’s type is different. A review consists of several pieces of information you need to keep track of, like the title, rating, and description.
As a result, you have to create a new data model called "Review" and link the review field within the User model to this new data type. This type is called a OneToOneField, because it links one field to another model. As a visual representation of this, you can see the arrow from the review field to the Review model above.
For information on all the categories and how to pick which one is right for your field, take a look here.
Attribute:
Attributes are characteristics about a particular field. For example, a CharField contains the following attributes:
"Null: true" means that Django will store empty values as NULL in the database if nothing is inputted. "Blank: true" means that the field is allowed to be blank. Finally, max_length determines the size of the database field that is used to store the data.
Adding Data Models to Apps
Your app’s data models are addable to apps within the app dashboard’s “Models” view. The Models section lets you add a list of models, specify fields for each model, and generate code with working APIs, database structures, and basic webpages that can be downloaded as a folder and then uploaded straight into a GitHub repo.
Each model should contain individual fields that correspond to the data records it will need to reference. Django automatically generates an “id” field that is used as the primary key. You can pick reasonable choices for each field’s data type: strings, characters, and so on.
Enter all of your models as described in the sections above. When you’re done, click “save” to ensure all your models are saved in your app.
Once you’ve uploaded your models, go ahead and click “Deploy App” on the Status page, then head over to the admin panel (found under "Settings" on the left-hand menu of the dashboard). Remember, credentials get emailed to the app’s creator, but you can change them once you go into the app admin panel.
Your app is now live with a working backend and a temporary frontend. You have the following three things available:
-
Admin panel containing all the models you created
-
Basic web views for all the models you created
-
User registration, user login, password resets
-
APIs for each model you created
If you make future changes to your models, make sure to not only save the page, but deploy your app again. Once you deploy, the changes will take effect.
Understanding the admin panel
Crowdbotics generates terrific admin panels that have a lot of handy built-in features. Here’s what you can do out of the box:
- Add and remove users
- Reset passwords
- Define which users have access to the admin panel (click the staff checkbox on a user’s account in the users list and save to give someone access)
- Create user groups (click on Groups, or click on a user and click + to create a group)
- Define which user groups have which permissions to access which models
- View all database objects that you’ve created
- Add and remove database objects individually
It’s easy to add or remove things to and from the admin panel, so we recommend using this as the main way to manage your database.
Automated API documentation
When you use the Model Builder, you’re actually creating cloud-based, secure, fully documented REST APIs through visual assembly. These APIs are generated in the background whenever you edit a data model.

The Crowdbotics Model Builder uses self-documenting API endpoints. This means that if you visit any URL for an API in a Crowdbotics app, it will show the automatic documentation for that API. This documentation includes the valid parameters you can submit and any notes from the comments in the code, saving you the effort of writing your own documentation after the fact.
This type of documentation comes directly from Django Rest Framework, so you will get it anytime you’re building backends with Django (even if you don’t use the Crowdbotics Model Builder).
Storing and manipulating data is the fundamental purpose of all apps. With the Crowdbotics model builder, you can create your app's foundation all on your own – no coding required.